Data structures are essential building blocks in computer programming. They are used to organize, store, and retrieve data efficiently. The efficiency of a program largely depends on the choice of data structures used in its implementation. In this blog, we will discuss what data structures are, their types, and their applications. This is just the overview of what data structures are. We will dive into each data structure individually in other posts.
What is a Data Structure?
In computer science, a data structure is a way of organizing data in a computer’s memory or disk storage. It provides a logical and efficient way of accessing and manipulating data. A data structure can be considered as a collection of values, with relationships among them, and operations that can be performed on them.
Types of Data Structures
There are several types of data structures, each with its own advantages and disadvantages. Some of the most commonly used data structures are:
- Arrays: An array is a collection of elements of the same data type. The elements are stored in contiguous memory locations and can be accessed using an index. Arrays are simple and easy to use, but their size is fixed and cannot be changed during runtime. Read more about arrays here.
- Linked Lists: A linked list is a collection of nodes, each containing a value and a pointer to the next node. Linked lists are dynamic and can grow or shrink during runtime. However, they require more memory than arrays, and their access time is slower.
- Stacks: A stack is a collection of elements arranged in a last-in-first-out (LIFO) order. Elements can be added or removed only from the top of the stack. Stacks are used to implement recursion, undo/redo functionality, and backtracking algorithms.
- Queues: A queue is a collection of elements arranged in a first-in-first-out (FIFO) order. Elements can be added at the rear end and removed from the front end of the queue. Queues are used in scheduling, resource allocation, and buffering.
- Trees: A tree is a collection of nodes, where each node has a value and zero or more child nodes. Trees are used in hierarchical data structures, such as file systems, organization charts, and decision trees.
- Graphs: A graph is a collection of nodes and edges, where each edge connects two nodes. Graphs are used in network analysis, social network analysis, and shortest path algorithms
Classification of Data Structures
Linear and non-linear data structures are two different ways of organizing data in computer programming. The primary difference between them is the way they store and retrieve data.
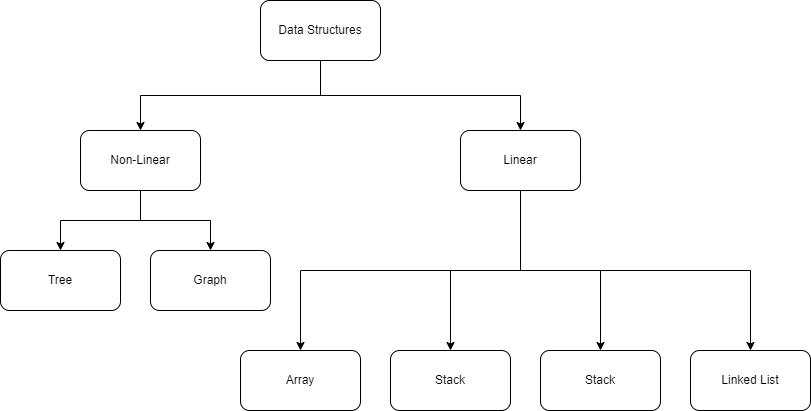
Linear Data Structures
Linear data structures store data in a sequential manner, with each element connected to its adjacent elements. The elements can be traversed in a linear manner, either from the beginning or the end. Examples of linear data structures include arrays, linked lists, stacks, and queues.
Arrays are a simple and commonly used linear data structure that stores elements of the same data type in a contiguous block of memory. The elements are accessed using an index, which is an integer value that represents the position of the element in the array.
Linked lists are another linear data structure that consists of a series of nodes, where each node contains a data value and a reference to the next node in the list. Linked lists are useful for dynamic data storage, as nodes can be added or removed at any position in the list.
Stacks and queues are also linear data structures, but they have specific access restrictions. Stacks follow a Last In First Out (LIFO) order, where the last element added is the first to be removed. Queues follow a First In First Out (FIFO) order, where the first element added is the first to be removed.
Non-Linear Data Structures
Non-linear data structures store data in a hierarchical manner, with each element connected to one or more child elements. The elements can be traversed in a non-linear manner, as there may be multiple paths to reach a particular element. Examples of non-linear data structures include trees and graphs.
Trees are a non-linear data structure that consists of a root node, which has one or more child nodes, each of which may have their own child nodes. Trees are useful for representing hierarchical data structures, such as organization charts and file systems.
Graphs are another non-linear data structure that consists of a set of nodes and edges that connect them. Graphs can be directed or undirected and can have cycles or be acyclic. Graphs are useful for representing relationships between objects, such as social networks and transportation networks.
Applications of Data Structures
Data structures are used in various applications, such as:
- Database Management Systems: Data structures are used to store and retrieve data efficiently in database management systems.
- Compilers: Data structures are used to represent the source code in compilers and to optimize the code generation process.
- Operating Systems: Data structures are used to manage processes, threads, and memory allocation in operating systems.
- Artificial Intelligence: Data structures are used in machine learning algorithms, such as decision trees and neural networks.
Conclusion
Data structures are essential tools for computer programmers to efficiently organize and manipulate data. The choice of data structure depends on the application requirements and the data being stored. Understanding the advantages and disadvantages of each data structure is important to select the right one for a particular application. Linear data structures store data in a sequential manner and can be traversed in a linear fashion, while non-linear data structures store data in a hierarchical manner and can be traversed in a non-linear fashion. The choice of data structure depends on the specific requirements of the application and the type of data being stored. Understanding the differences between linear and non-linear data structures is essential for efficient data storage and retrieval in computer programming.
0 Comments